Common Instructions
Objective
Complete the Bubbl Plugin implementation with the common steps for both manual and Cocoapods integration.
Audience
iOS App Developer
Common instructions for both Manual and Cocopods installation methods
Prepare Capabilities
Select your project in the navigation pane:
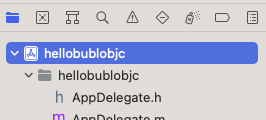
Then select your projects target:
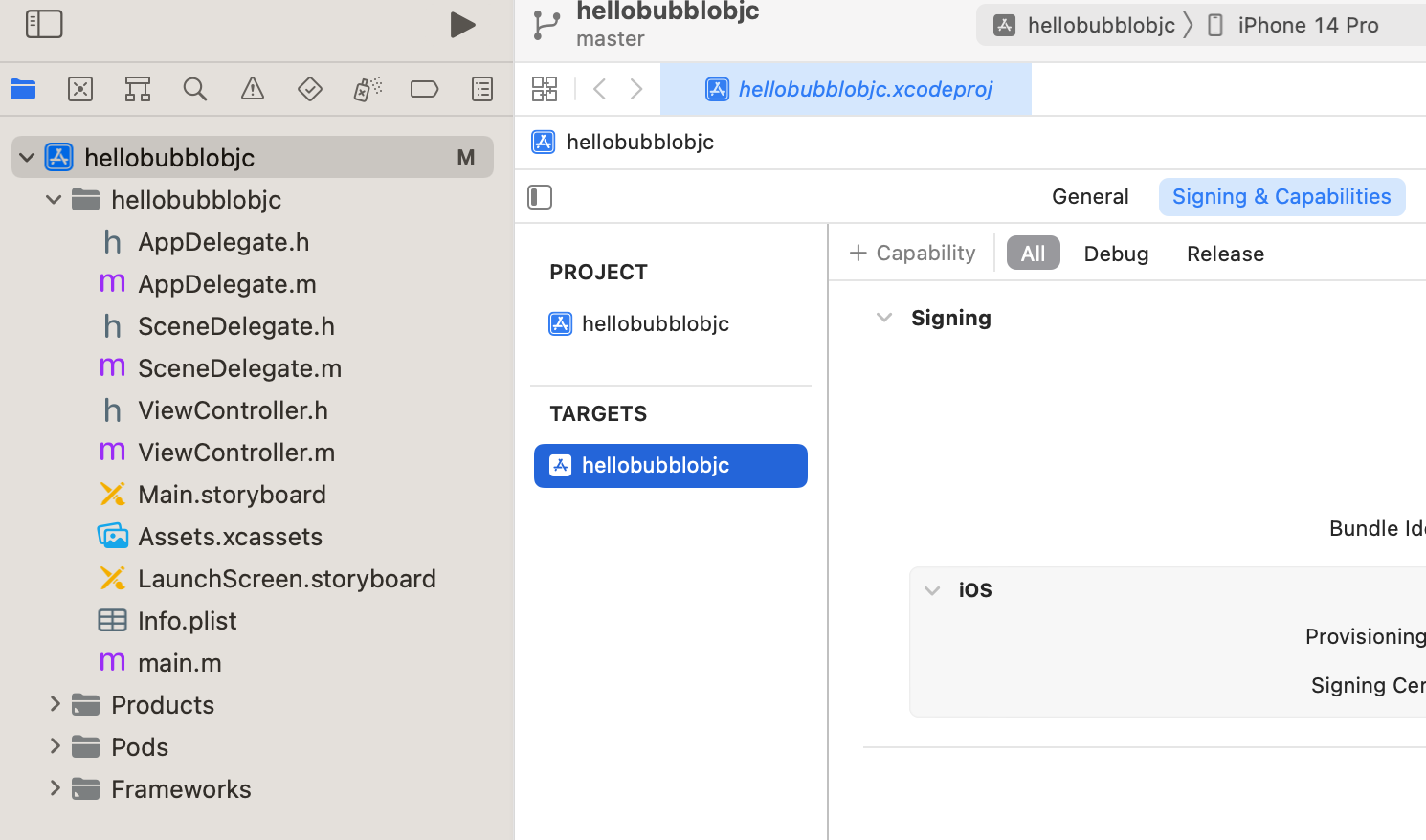
Background Modes
For Bubbl to work correctly the following Background Modes need to be enabled.
If you do not currently have Background Modes enabled click the + Capability
button.
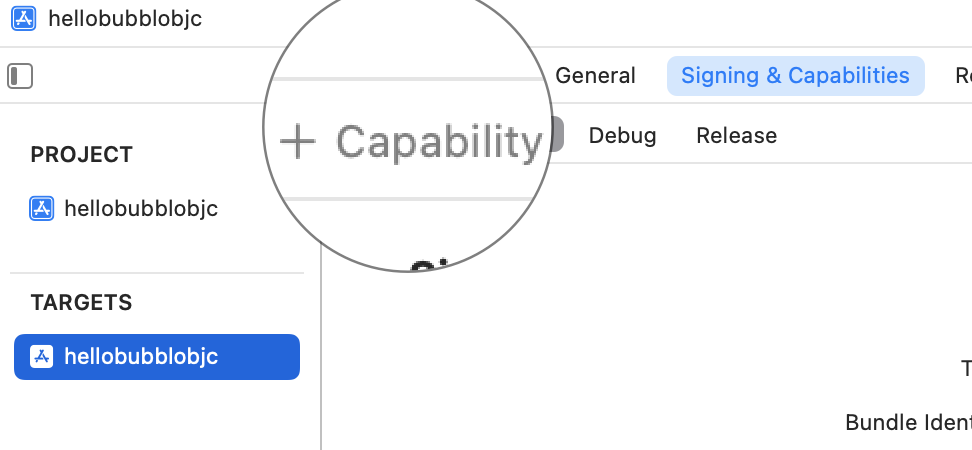
And select Background Mode
.
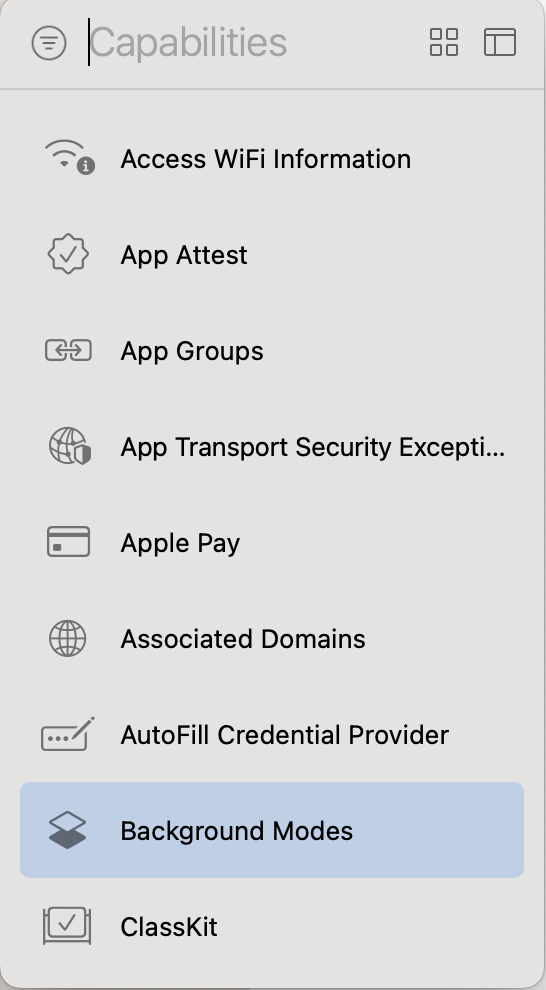
The following Background Modes need to be enabled:
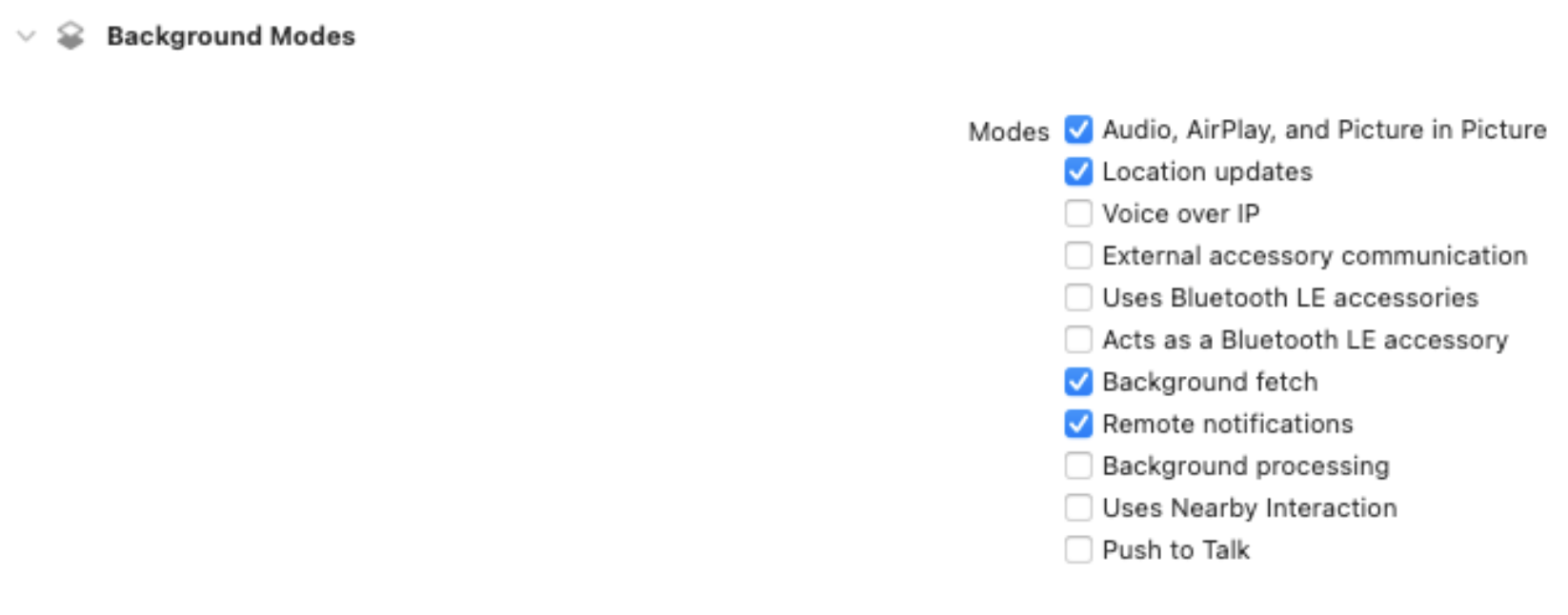
Enable Push Notifications
Click the + Capability
button again if you do not currently have Push Notifications enabled.
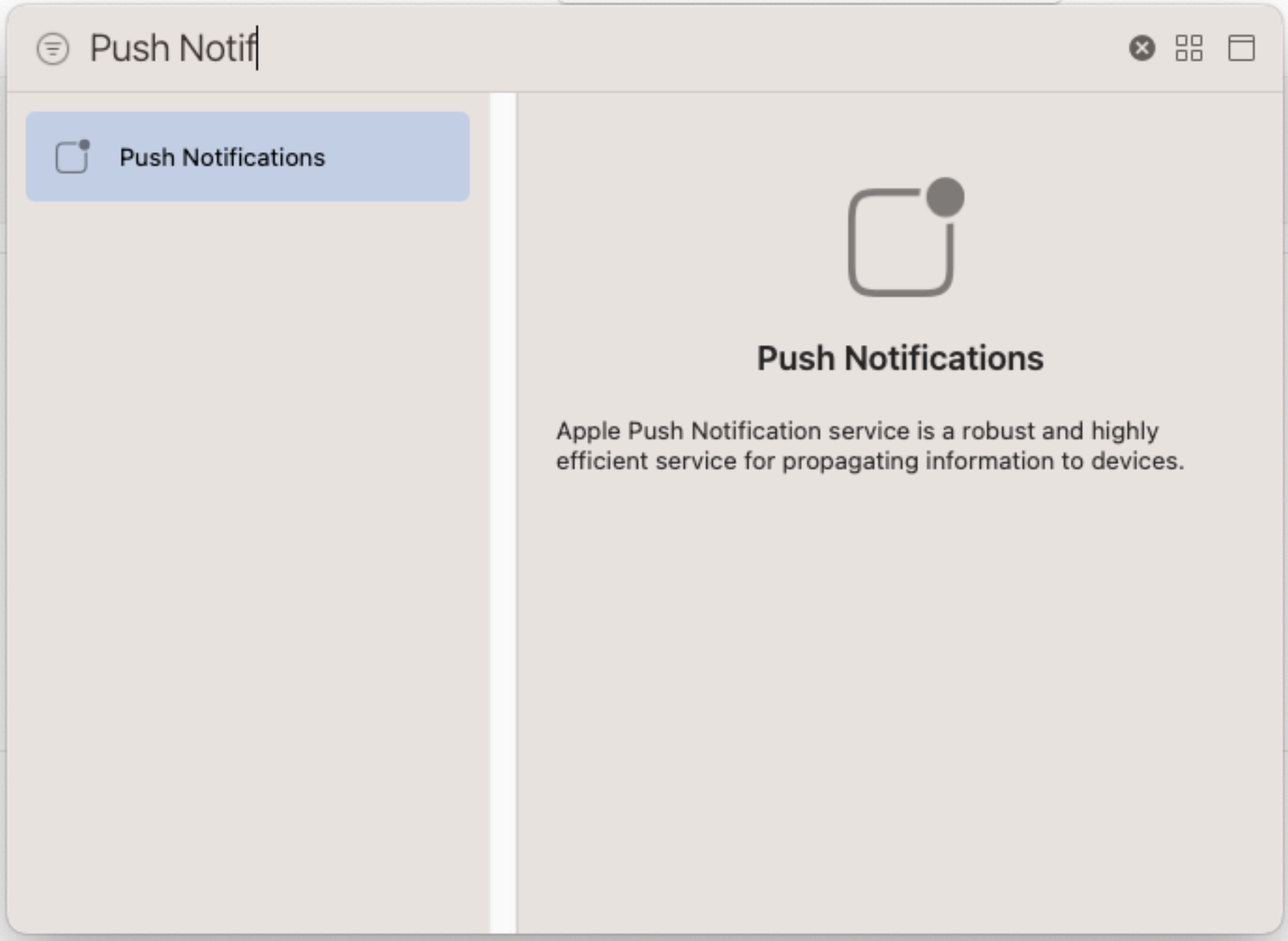
Enable App Group
To enable App Groups, click the โ+ Capabilityโ button in the toolbar and add โApp Groupsโ
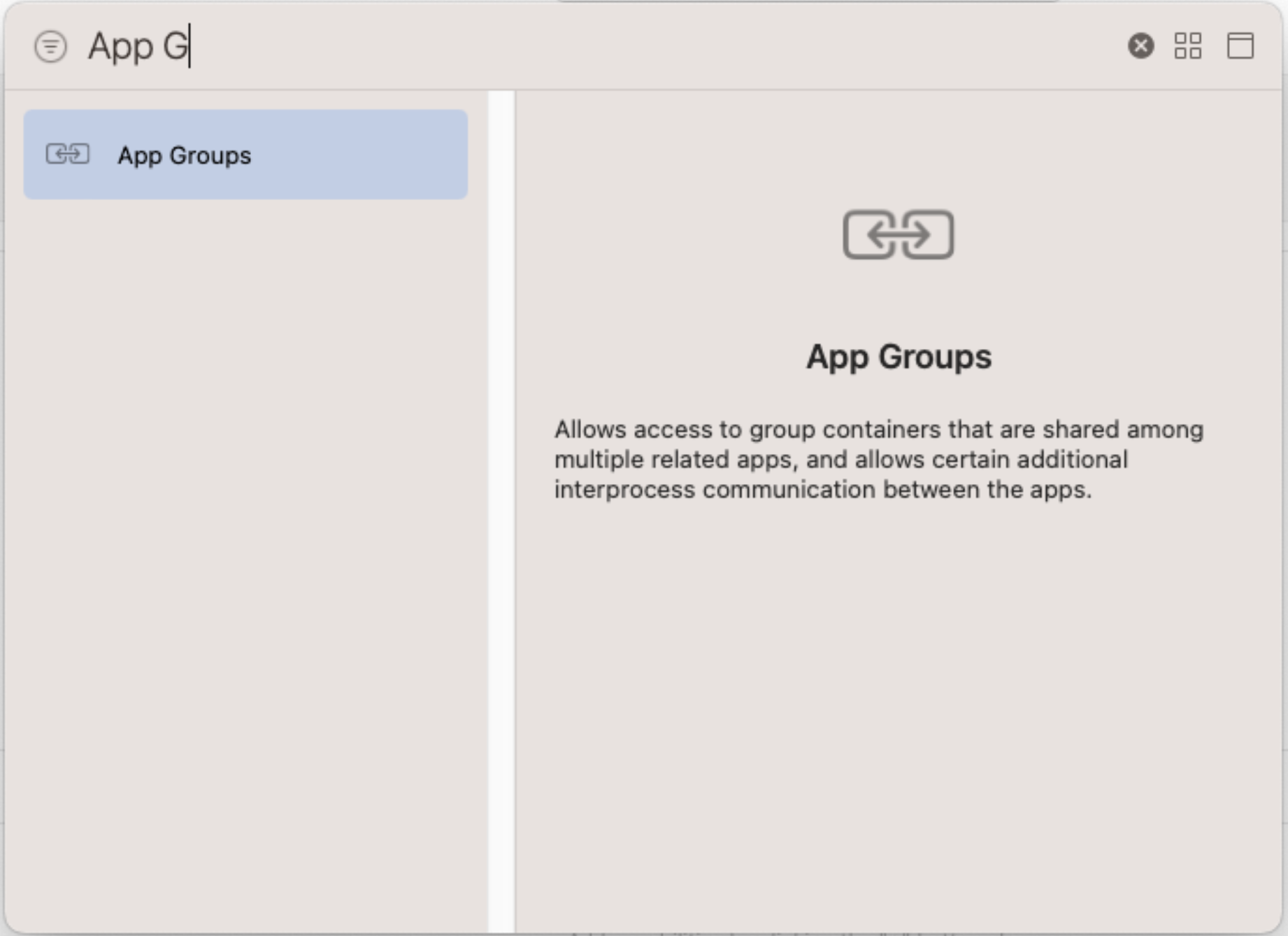
Click the โ+โ button under App Groups to add a new app group:
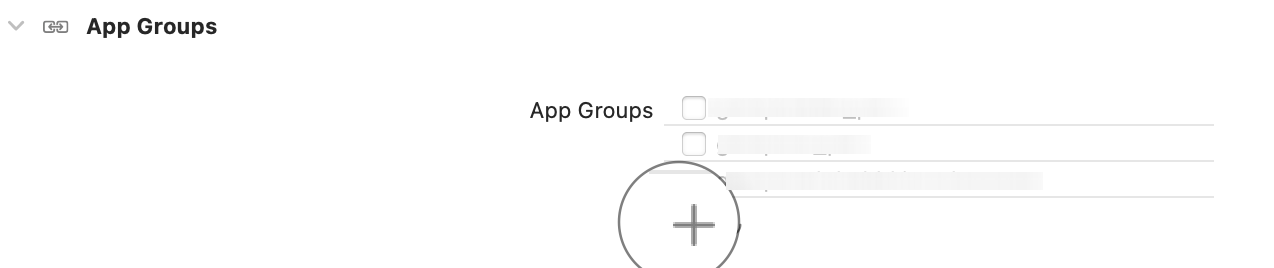
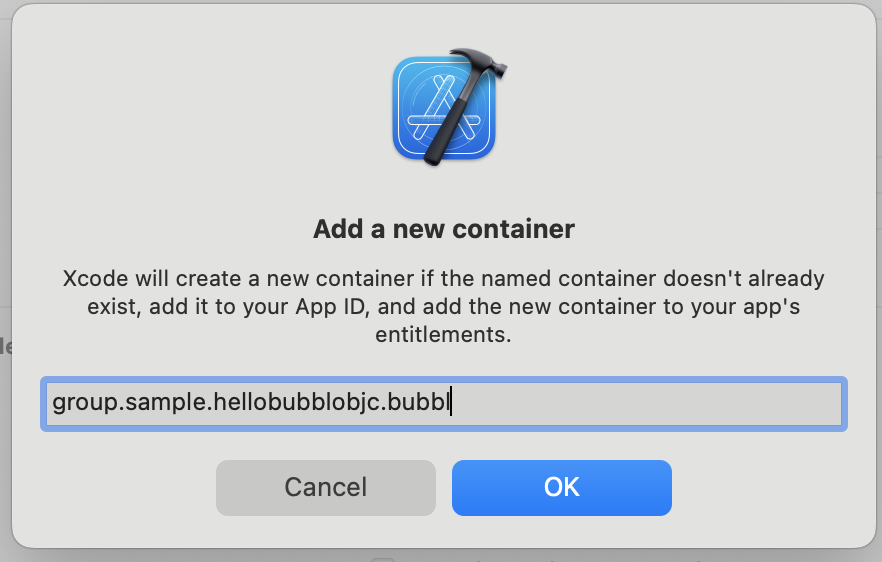
It is recommended to give it a name starting with โgroup.โ followed by the bundle identifier of your application and suffixed with โ.bubblโ. This App Group will be required for setting up the plugin.
Include Frameworks
Select your project in the navigation pane and select the General tab:
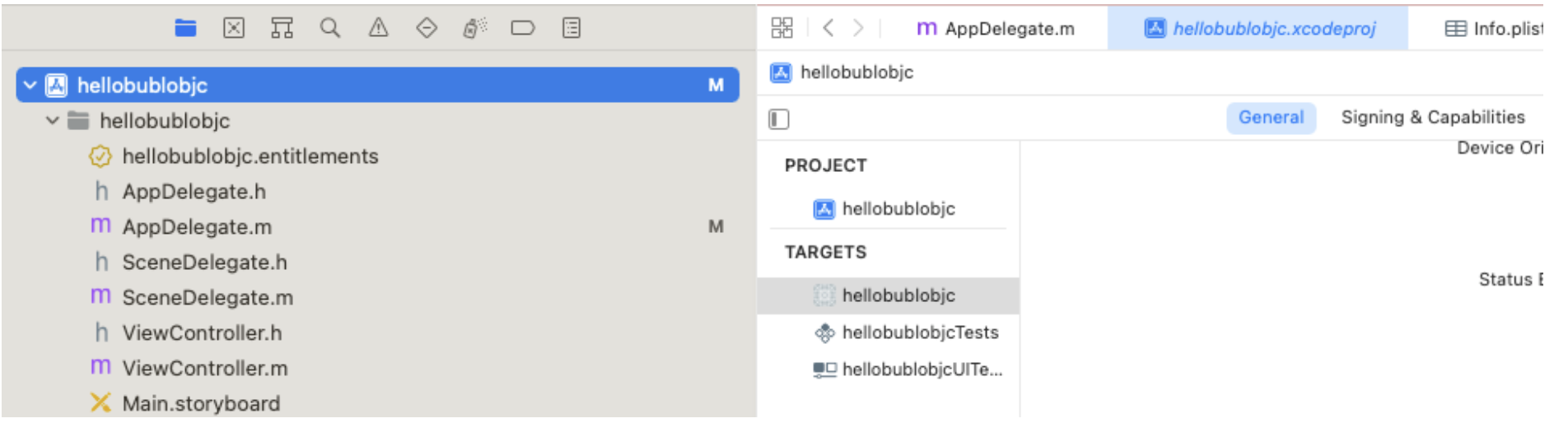
And add the following 3 Core Frameworks by clicking on the +
button.
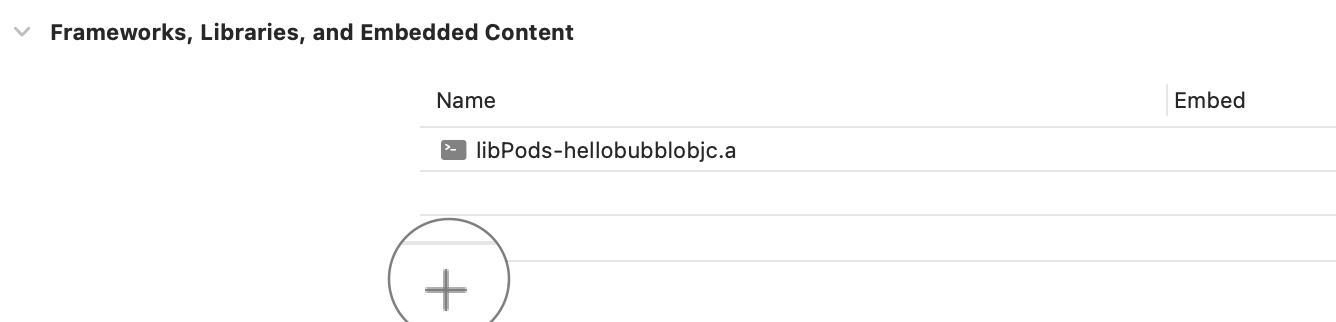
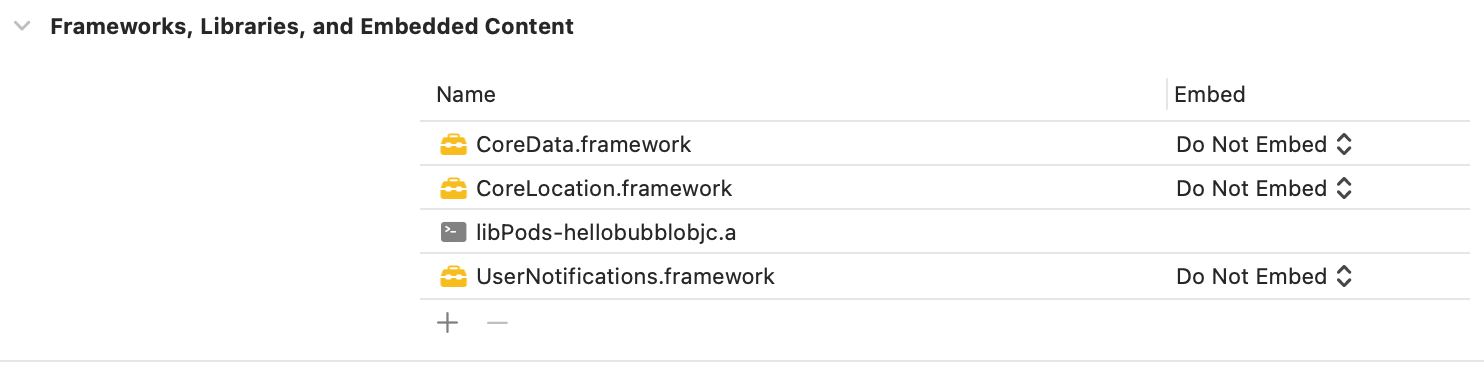
Add a Notification Service Extension
For Bubbl to perform most of the important features such as displaying media within notifications, showing badges to indicate unread notifications, adding Call to Action buttons or reporting notifications as delivered we must have a notification extension present in your application.
To create a notification extension add a new Target using the File menu:
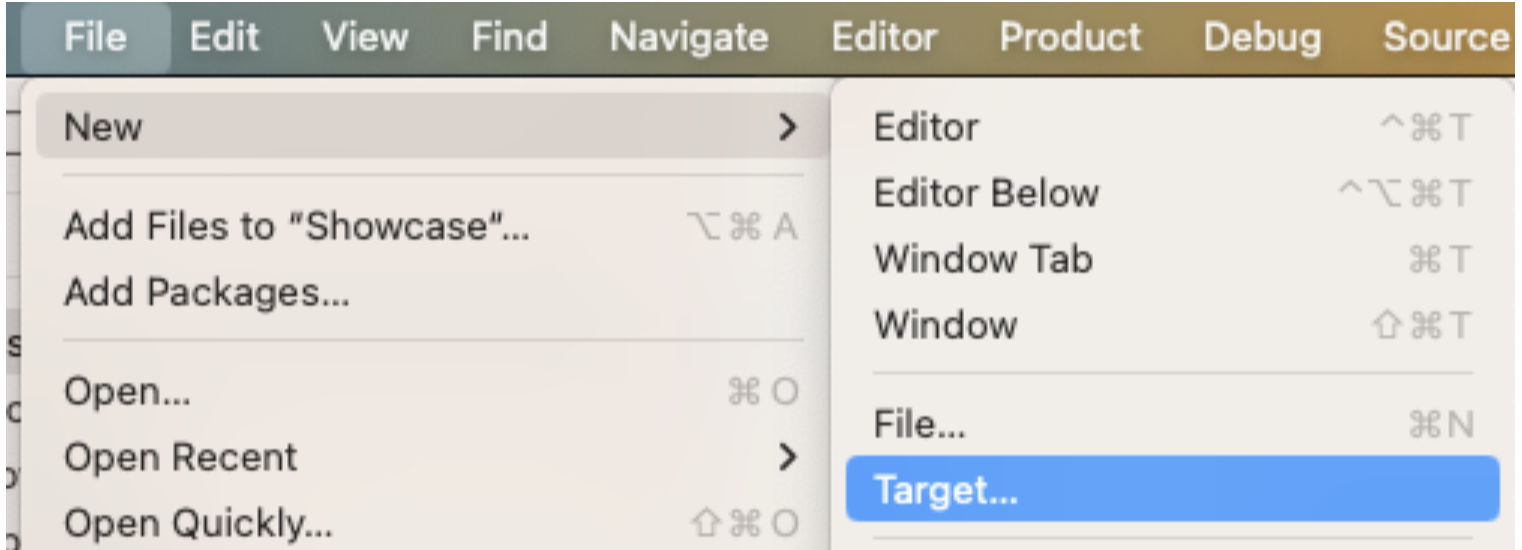
Select Notification Service Extension:
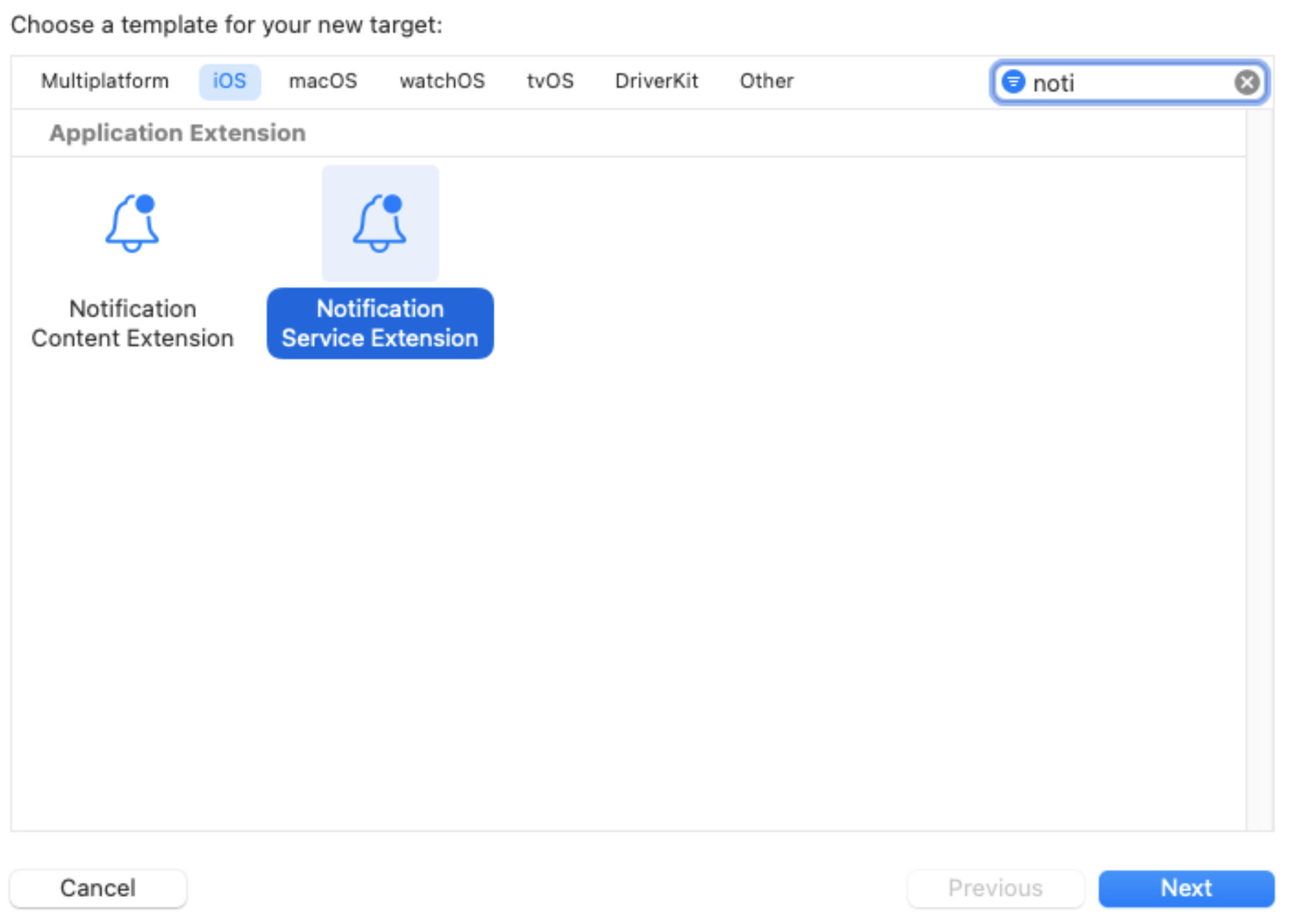
Add the Notification Service Extension. The name can be anything you would like, but ensure it is added to the correct project and embedded in the correct application:
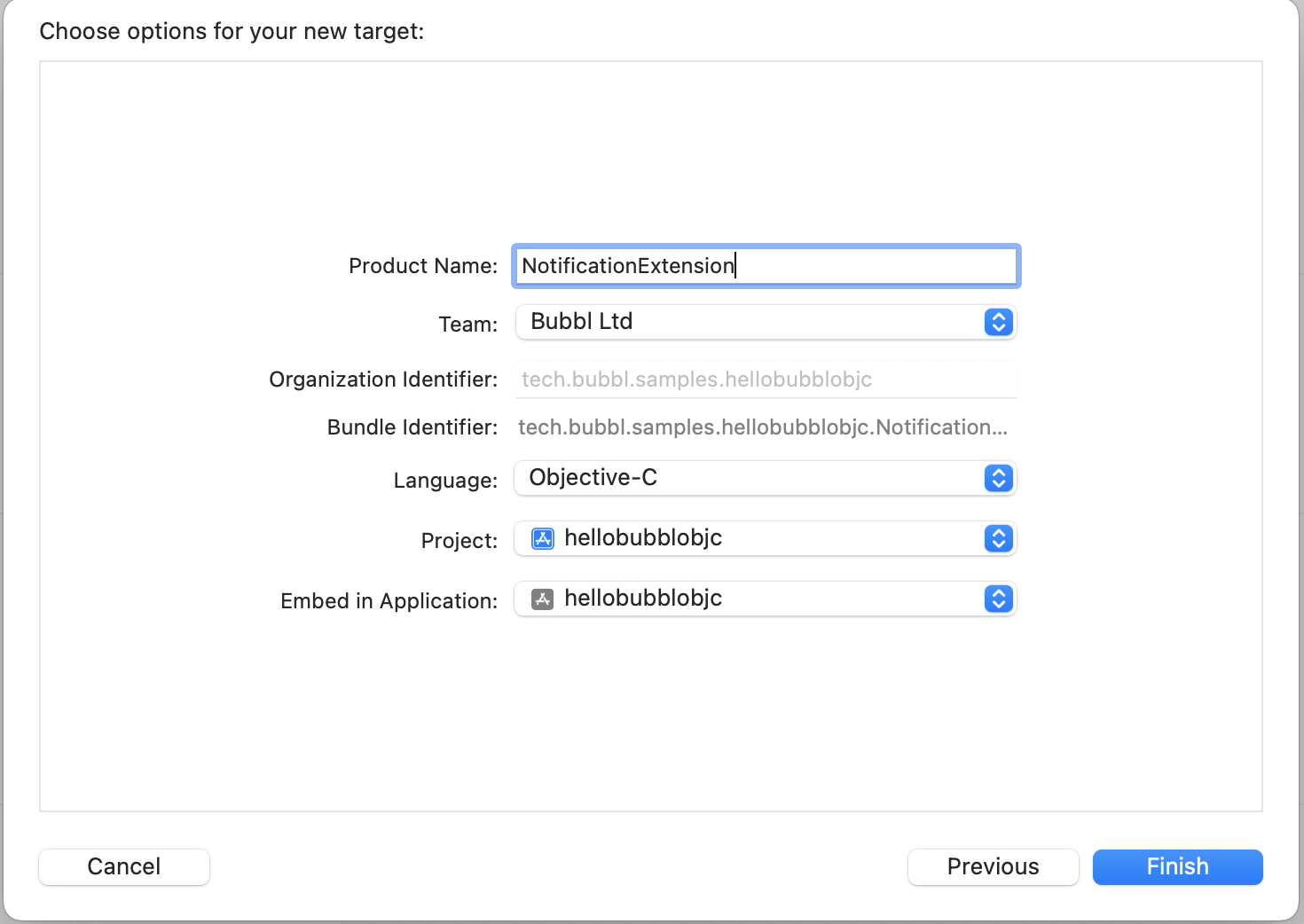
Upon clicking finish you should be prompted to activate the extension, click โActivateโ
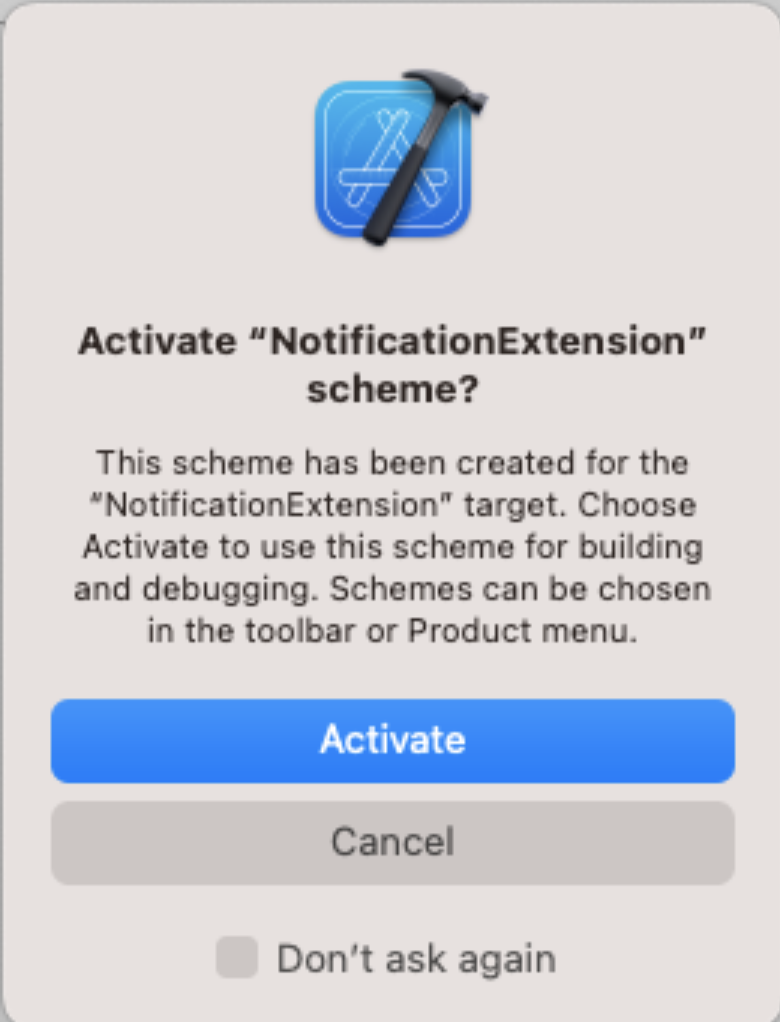
Under the Notification Extension settings ensure you add the โApp Groupsโ capability, with the same App Group as your application:
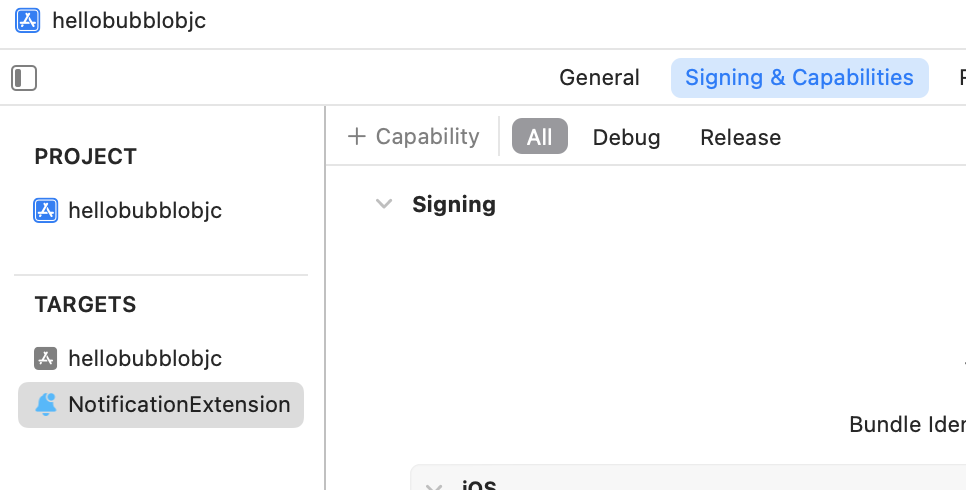
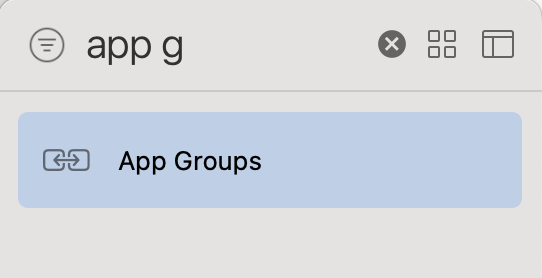
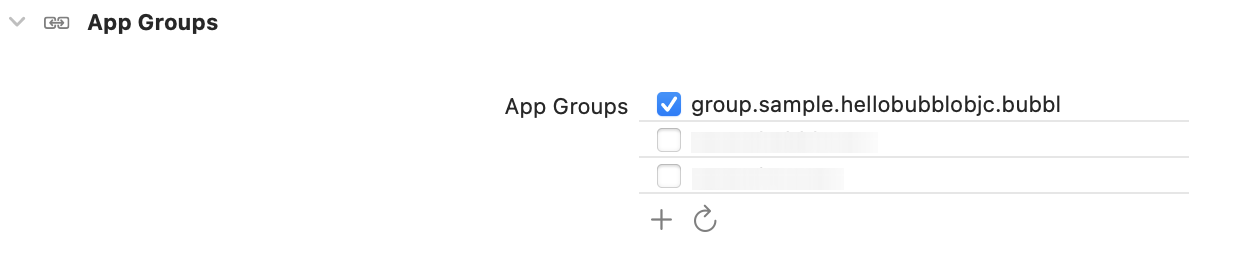
Under General you will need to add the Bubbl framework.
Do this by clicking the +
on Frameworks and Libraries
.
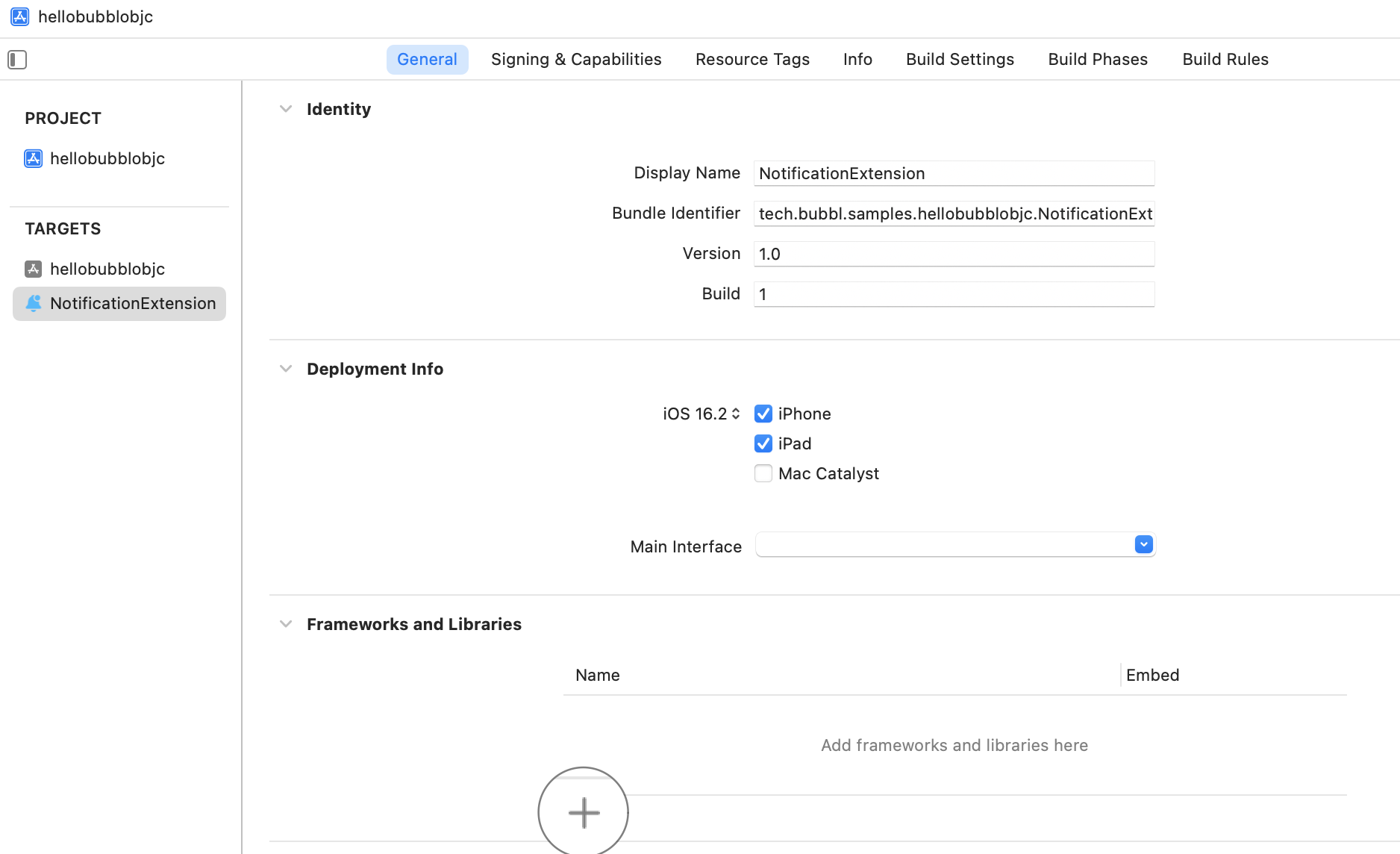
Select Add Files...
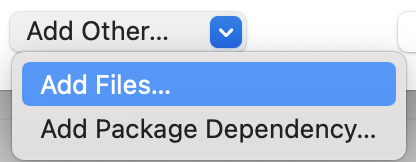
Navigate up one level in the directly hierarchy.
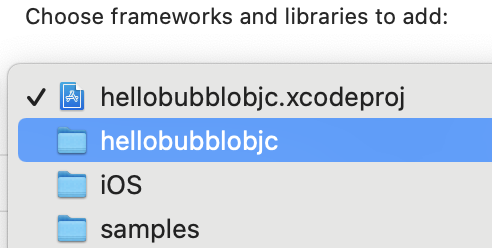
And select Bubbl.xcframework
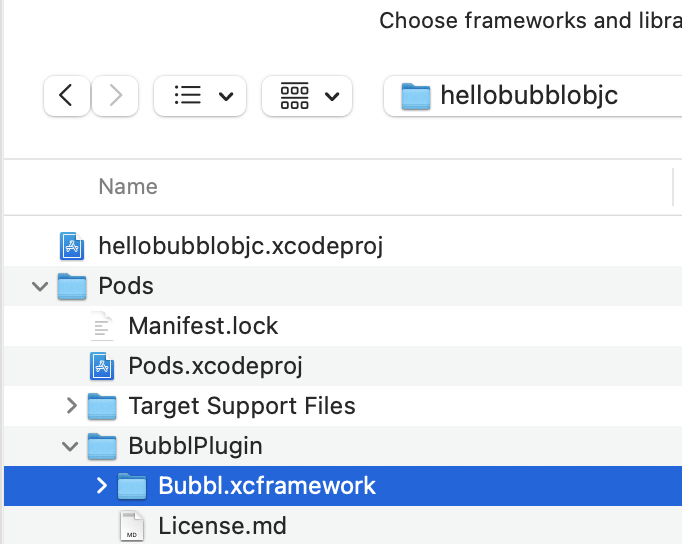
Change the Embed
value from Embed & Sign
to Do Not Embed

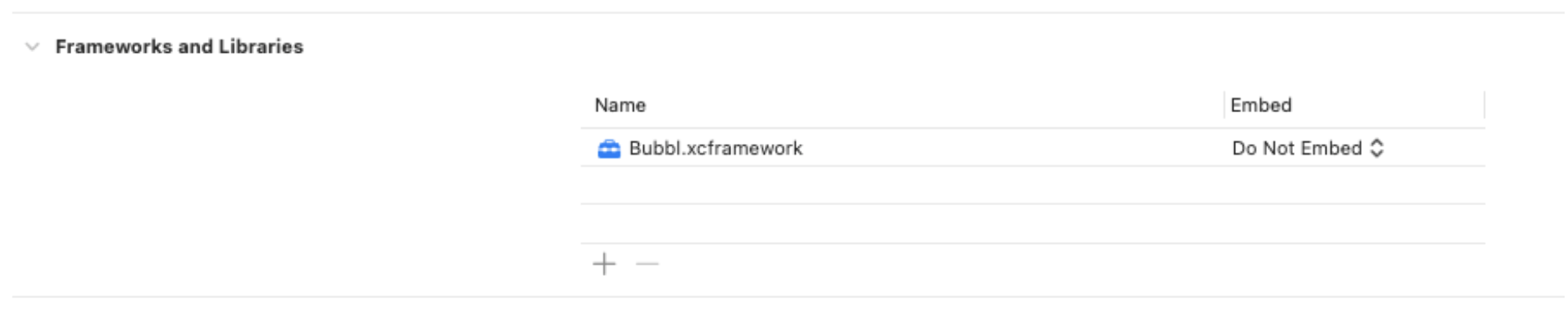
There should now be a few files added to your project for your notification extension, we need to modify these files. They may be either in Swift or Objective C depending on the language choice when setting up.
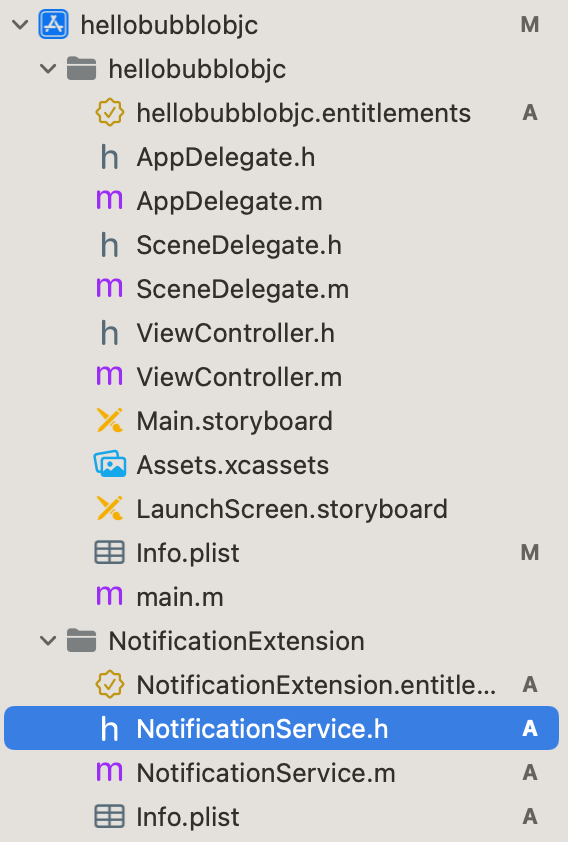
The Notification Service class that was just created should extends BUNotificationService
extension:
#import <UserNotifications/UserNotifications.h>
#import <Bubbl/Bubbl.h>
@interface NotificationService : BUNotificationServiceExtension
@end
The class should register the App Group in the initializer:
@implementation NotificationService
// on init set the app group identifier in the base class
- (instancetype)init {
self = [super init];
if (self) {
self.appGroupIdentifier = @"<APP GROUP HERE>";
}
return self;
}
@end
import UserNotifications
import Bubbl
class NotificationService: BUNotificationServiceExtension {
override init() {
super.init()
setAppGroupIdentifier("<APP GROUP HERE>")
}
}
Troubleshooting
Ensure that the iOS deployment target is the same version as your application, otherwise the Notification Extension may not run on older devices.
Adding the Plugin
Your AppDelegate should be updated to setup the Bubbl plugin the didFinishLaunchingWithOptions method, and we also need to inform the plugin when a device token is received or notifications will be presented or responded:
Add the import for Bubbl to the AppDelegate.h
so that it can be used in the AppDelegate.m
class.
#import <Bubbl/Bubbl.h>
#import <Bubbl/Bubbl.h>
@interface AppDelegate () <UNUserNotificationCenterDelegate>
@end
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Provide the API key and the endpoint URL to connect to
[BUMain setupWithKey:@"<YOUR API KEY>" withURLString:@"https://mobilegateway.bubbl.tech/api" withAppGroup: @"<APP GROUP HERE>"];
// provide additional debugging information
[BUMain sharedInstance].debugMode = YES;
// register for notifications
[application registerForRemoteNotifications];
[UNUserNotificationCenter currentNotificationCenter].delegate = self;
return YES;
}
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken {
[BUMain registerForRemoteNotificationsWithDeviceToken: deviceToken];
}
- (void) userNotificationCenter:(UNUserNotificationCenter *)center willPresentNotification:(UNNotification *)notification withCompletionHandler:(void (^)(UNNotificationPresentationOptions))completionHandler {
[[BUMain sharedInstance] userNotificationCenter:center willPresentNotification:notification withCompletionHandler: completionHandler];
}
- (void) userNotificationCenter:(UNUserNotificationCenter *)center didReceiveNotificationResponse:(UNNotificationResponse *)response withCompletionHandler:(void (^)(void))completionHandler {
[[BUMain sharedInstance] userNotificationCenter:center didReceiveNotificationResponse:response withCompletionHandler:completionHandler];
}
@end
import UIKit
import Bubbl
@main
class AppDelegate: UIResponder, UIApplicationDelegate, UNUserNotificationCenterDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
// Provide the API key and the endpoint URL to connect to
BUMain.setup(withKey: "<YOUR API KEY>", withURLString: "https://mobilegateway.bubbl.tech/api", withAppGroup: "<APP GROUP HERE>")
// provide additional debugging information
BUMain.sharedInstance().debugMode = true
// register for notifications
application.registerForRemoteNotifications()
UNUserNotificationCenter.current().delegate = self
return true
}
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
BUMain.registerForRemoteNotifications(withDeviceToken: deviceToken)
}
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
BUMain.sharedInstance().userNotificationCenter(center, willPresent: notification, withCompletionHandler: completionHandler)
}
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
BUMain.sharedInstance().userNotificationCenter(center, didReceive: response, withCompletionHandler: completionHandler)
}
}
Permissions descriptions
Your application's descriptions for the following permissions need to be updated with using XCode or by updating the Info.plist:

...
<key>NSLocationAlwaysAndWhenInUseUsageDescription</key>
<string>Your message here</string>
<key>NSLocationAlwaysUsageDescription</key>
<string>Your message here</string>
<key>NSLocationWhenInUseUsageDescription</key>
<string>Your message here</string>
<key>NSPhotoLibraryUsageDescription</key>
<string>Your message here</string>
...
An example message could be something like To provide a tailored and personal service we need to have access to your location
.
Updated almost 2 years ago